CSS, short for Cascading Style Sheets, is a key tool for web designers. In this intro to CSS, we'll cover the highlights of what you should know about this language. But before diving in, you should know the pains developers went through before CSS. In the past, we styled an application directly in our markup, which was very difficult to maintain. This was one of the first problems CSS aimed to solve: by decoupling styles from content, we gained the power to update the presentation for many pages all at once.
In the early days, CSS was full of hacks to account for bugs in rendering engines and to support various browsers with inconsistent implementations of the CSS specifications. This has led to CSS having a poor reputation as a hacky, legacy, and arcane language.
However, we currently live in the golden age for CSS. It's now a mature language that is still growing and evolving behind the scenes.
With this intro to CSS, you'll gain an understanding of the role CSS plays in a web application, how CSS applies styles, and a breakdown of the most frequently used concepts with their related properties.
It takes time to master CSS, but with this article guiding you, you'll be well on your way to becoming a master.
In this article
The 4 layers of a web application
A web application is comprised of four parts:
- Business logic
- Content (HTML)
- Interaction (JavaScript)
- Presentation (CSS)
Business logic is the most ambiguous, so for this article, we'll assume that it's server-side code, or code that interacts with a server.

Each time a web page is requested by a browser, the business logic sends down HTML, which we'll call content. This content is unstyled black text on a white background at this point in time.
As the HTML is loaded, the browser will load more assets, like images and videos, but more importantly, JavaScript and CSS.
JavaScript can do many great things because it's a general purpose programming language. At the core, in a web browser, JavaScript is used to handle user interaction. For example, if a user clicks a button, something happens. This "something" is everything from submitting a form to changing the HTML document.
CSS uses the structure of the HTML to style the webpage. When the CSS code is read, the content transforms from black text on a white background to whatever creation has been described by the collection of styles.
To see some examples of the raw power of CSS, please explore the examples on CSS Zen Garden.
Going under the hood of CSS
The foundation of writing scalable CSS for our web application is a ruleset. A ruleset contains one or more selector(s) and a declaration block with a collection of declarations. Declarations contain properties and values in a key/value pair syntax.

Selectors are the interface that CSS uses to match our rulesets to elements in our HTML document. Multiple selectors and rulesets might apply to the same element, and this is where the 'cascade' part of CSS comes into play.
The cascade is the referee that determines which properties are applied to a given element, with considerations to the specificity of the selector and possible inherited properties. We need a referee to determine which styles take precedence in the event of a rule conflict.
As a rule of thumb, the cascade determines which properties apply in this order:
- Level of selector specificity
- Source order of rulesets
- Inherited values
For an in-depth approach to the cascade, check out this MDN article on the cascade and inheritance, or to go even deeper, read the latest spec on cascading and inheritance.
For an introduction on just the topic of specificity, CSS Tricks has an excellent article called the Specifics on CSS Specificity.
Knowledge check-in
At this point, you should have a high-level understanding of CSS, its role in web applications, and how styles are applied to elements by the browser. What you might be wondering is how to actually style content. In the next section of our intro to CSS, you'll learn about some properties and how they help build awesome visual experiences for web pages.
You might also like: A Modern Approach to CSS Pt. 1: Tools and Organization.
The basics of styling a web application
Before getting into the meatier concepts of CSS, let's cover a few fundamentals when it comes to styling and rendering content.
1. The concept of rectangles
When styling content, there are a few things to keep in mind to help build a solid mental model of how our content will be rendered:
- Everything is a rectangle. Even if it doesn't look like a rectangle, it's bounded by one.
- Every rectangle relates to another rectangle in some way or another.
- Nested (child) rectangles are above their containing (parent) rectangles by default.
Setting these guidelines will help you when you are laying out web pages.
2. The display
property
The first property we should learn about in this intro to CSS is the display
property. There are a handful of different values for display
, and we'll cover some of them below, but the two we should be aware of right now are block
and inline
. All HTML elements by default will have one of these two values.
Block-level elements will be rendered from the top to bottom of their parent rectangle, with each block-level sibling starting below the previous element.
Think about a heading, followed by a paragraph, another heading, and then a final paragraph. We view these elements from top to bottom.
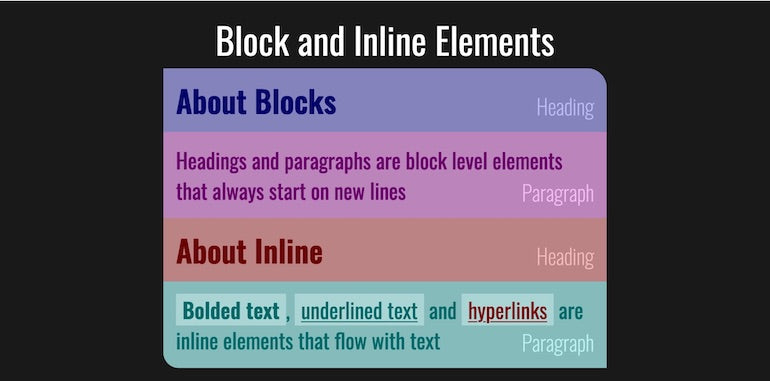
Inline elements will be rendered from left to right (or right to left for languages natively read this way), with their contents wrapping to the next line and their next inline sibling starting after the previous content.
Think about some bold text, followed by underlined text, a link, and then some normal text. We view these elements in a reading direction and then top to bottom.
You can read more about block-level elements and inline elements in Mozilla's web docs. At the bottom of each of those pages are a list of all elements with their respective default display property.
3. Document flow
The basics of document flow are how block-level and inline elements are rendered. The important thing to know is that rectangles are placed from top to bottom or in a reading direction, for each display type respectively.
However, there are times when we don't want our content to follow this straightforward approach to document flow, so CSS provides different ways to manipulate it. We'll cover those specifics later on but for now, just being aware of this possibility is good enough.
To get a better understanding of document flow, you can read this article on In Flow and Out of Flow.
4. Typography
The foundation of the web is content, and the simplest form of content is text. When working with typography on the web, there are several things you need to keep in mind to make your designs practical and appealing.
For readability and accessibility, we need to consider the contrast of text color to the background color and the size of the font depending on the font family. For pixel perfection, it's important to know that line-height
controls line spacing while font-size
has less influence.
See the Pen Demo: Typography by Tyler Childs (@tylerchilds) on CodePen.
Related properties
Here are a list of related properties to be familiar with:
-
font-family
: A prioritized list of fonts, where the first font available will be used -
color
: The color of the text -
text-align
: The alignment of the text in the element -
font-size
: The height of the text itself -
line-height
: The height of the line of text, where the invisible space is based on this andfont-size
-
font-weight
: The weight of the text, such as bold -
font-style
: The style of the text, such as italic -
text-decoration
: The decoration of the text, such as underlined -
letter-spacing
: The space in between letters -
word-spacing
: The space in between words -
text-shadow
: The shadow effects around the text
Note: All of these typography-related properties will inherit, meaning that if we set them on a parent ruleset, they will apply to all their descendants.
5. The box model
The most fundamental aspect of styling rectangles is the box model, which is concerned with the properties associated with every rectangle. At the core of the box model is content, which is either a child rectangle or an empty rectangle. Around the content is padding, followed by border, and finally margin, just like rectangles inside more rectangles. We'll now cover more details of the model.
box-sizing
The box-sizing
property controls how the box model is calculated. There are two primary values for box-sizing
: content-box
and border-box
. content-box
is the default for all elements.
content-box
calculates the box model for a rectangle by adding padding and borders to the width and height of our content. content-box
is a pitfall for many, since it allows elements with a 100 percent width to become larger than 100 percent due to padding, borders, and margin.
border-box
calculates the box model by absorbing the border and padding for an element into the width and height. So, a 100 percent width element will be 100 percent including padding and border, but margin could still make the element larger than 100 percent width. If our margin is making our element overflow, we should consider adding padding or margin to the parent instead.
See the Pen Demo: Box Model by Tyler Childs (@tylerchilds) on CodePen.
Related properties
Here are several related properties for the box model:
-
width
: The width of an element* -
height
: The height of an element* -
max-width
: The maximum width of an element* -
max-height
: The maximum height of an element* -
padding
: The space around the element's content (Note: Setting a background paints the padded space of an element) -
border
: The border surrounding an element's padded space -
border-radius
: The roundness of any given corner of an element -
margin
: Empty space surrounding an element -
outline
: Additional border-like property that does not affect document flow or the box model size -
outline-offset
: Distance from the border of an element to the start of the outline -
box-shadow
: Shadow effects in or around an element
* With respect to box-sizing
Box model challenges
There are two main challenges when updating box model-related properties that you should keep in mind:
- Inline elements ignore the margins.
- Shared top and/or bottom margins between elements can 'collapse' and use only the largest value. You can read more about margin collapsing to find out why.
6. Layouts
After getting the hang of the box model, the next step in our intro to CSS is building layouts. The two most practical approaches to building layouts are by using Flexbox (Flex) or Grid, which are both display properties. Both flex
and grid
affect the positioning of child elements, and while there are many similarities between the two, they serve different purposes.
Both are extremely in-depth topics in their own right, so the best way to learn is by experimenting. Flexbox Froggy is a game to learn Flexbox by placing frogs on lilypads, while Grid Garden helps teach CSS Grid by working on a garden.
Beyond Grid and Flex, it’s also worth mentioning direction and writing modes. Direction controls if text should be written from left to right or right to left, which is inline direction. Writing modes go one step further and can control both block flow direction as well as inline direction. More detailed information can be found in this article on CSS Writing Modes.
You might also like: Embrace Localization and Multilingual Content with HTML and CSS.
Related properties
Here are several important related properties to keep in mind for layouts:
-
display
: Determines the block formatting context for an element and its children -
place-content
: Sets the space between and around content -
place-items
: Sets theplace-self
property for all direct children -
place-self
: Positions content relative to its respective axes -
gap
: Sets the gutters between rows and columns -
overflow
: Controls how content that is larger than an element should be handled -
direction
: Sets the direction text will flow, such as Left to Right (LTR) or Right to Left (RTL) -
writing-mode
: Controls block and inline content direction
Note: The place-* properties are used for both Flex and Grid, but are ignored for other display
values.
7. Stacking
Beyond layouts where everything follows the standard document flow, it’s possible to stack elements on top of each other. The foundation of this is the Stacking Context, which is formed whenever an element has certain properties, such as a position value other than static or a child of a grid or flex container.
Related properties
Here are some important related properties to keep in mind for stacking:
-
position:
: Determines the context in which an element can be positioned against -
top
,right
,bottom
,left
: Applies nudged positions relevant to the current stacking context -
z-index
: Specifies the stacking layer along the z-axis, higher values on top of lower values -
mix-blend-mode
: Mixes stacked layers with composite lighting effects
Note: The z-index
value of an element only has an effect in its respective stacking context and not all stacking contexts. This means that sometimes a higher z-index
value will be below a lower value, because it’s in a different stacking context.
8. Multimedia
When placing images and videos into a webpage with <img>
and <video>
tags, they will display inline by default. Floating an element will allow other content to wrap around it. To prevent an element’s contents from wrapping around a floated element, it can be cleared.
To size an image or video relative to its container, object-fit
provides similar values as background-size
, while cover
and contain
both maintain aspect ratios. cover
will fill the container with the object and clip along the greater dimension, while contain
will show the entire object with empty space along the smaller dimension.
Related properties
Here are some important related properties to keep in mind for multimedia:
-
float
: Removes an element from document flow, allowing content to flow around it -
clear
: Disregards previously set floats -
object-fit
: Determines how media objects should fit in their containing element -
background
: Sets background images or colors for an element -
background-size
: Determines how backgrounds should fit in their element -
clip-path
: Allows for the masking of an element’s contents -
shape-outside
: Allows for a custom shape to bound an element
9. Transformations
After getting the hang of layouts and stacking, transformations are incredibly useful as a next step in your intro to CSS. They enable us to manipulate content in various ways without altering its position in the document flow. For example, when hiding an element by changing the opacity to 0, it’s still there, but totally invisible.
transform
allows for elements to be translated, skewed, rotated, and scaled. It also enables elements to be transformed in three dimensions (3D). This requires a parent element to have the perspective
property set, which essentially serves as a viewport into 3D Space.
See the Pen Demo: Transform by Tyler Childs (@tylerchilds) on CodePen.
Related properties
Here are some important related properties to keep in mind when it comes to transformations:
-
opacity
: Controls the transparency of an element and its children -
transform
: Modifies the coordinate space of the CSS visual formatting model -
transform-origin
: Sets the point for transformations to be applied around -
transform-style
: Controls if 3D elements should overlap or intersect -
perspective
: Enables the viewing of 3D elements and sets a viewing distance -
perspective-origin
:Changes the vanishing point for a 3D element -
backface-visibility
: Allows the back of an element to be visible and mirrored
Note: opacity
and transform
are the most performant properties to animate. Some information about that can be found in this article on rendering performance and a useful cheat sheet is CSS Triggers.
10. Transitions and animations
To get a slick user experience, animations can add a subtle and polished touch to your website. Animations can also be used together across a collection of properties, so content can be translated, scaled, and faded in harmoniously.
On the other hand, transitions are useful for transitioning a property from one value to another value, such as the background of a button getting darker when the user hovers over it with the mouse.
Animations are built using the @keyframe
syntax which allows us to add rulesets using percentages. When the animation is applied using the animate
property, each of those rulesets will be triggered in order based on the duration.
See the Pen Demo: Animations by Tyler Childs (@tylerchilds) on CodePen.
Related properties
Here are some important related properties to keep in mind when it comes to transitions and animations:
-
transition
: Controls how properties should change when their values update -
transition-delay
: Controls the delay before a transition begins -
transition-duration
: Controls the length of time of a transition -
transition-property
: Controls the property to be transitioned -
transition-timing-function
: Controls the easing function to use for a transition -
animation
: Applies fine-tuned keyframe animations to a ruleset -
animation-delay
: Controls the delay before an animation begins -
animation-direction
: Controls how an animation traverses keyframes -
animation-duration
: Controls the length of time of an animation -
animation-fill-mode
: Controls where an animation should rest when completed -
animation-iteration-count
: Controls how many times an animation should repeat -
animation-name
: Controls the name of the animation declared using@keyframes
-
animation-play-state
: Controls whether an animation is paused or running -
animation-timing-function
: Controls the easing function to use for an animation
11. Conditional design
While CSS doesn’t have typical control flow like other programming languages, it does have some conditional “at-rules”. These enable you to specify nested CSS rulesets to be applied if the given conditions are met. Two examples are:
-
@media
: With regards to responsive design we have @media, which enables us to check the browser’s window size, screen pixel density, aspect ratio, and the device’s media type. -
@supports
: Since CSS is an ever-evolving language, not all browsers support the latest CSS features. While new properties won’t break CSS if the browser can’t understand them, we can wrap relevant rulesets into @supports to check if a feature exists to keep our code more explicit and organized.
From foundation to mastery
First off, I just want to thank you for following along. I know this article is technically dense, but you got through it and now have a solid foundation in CSS. We’ve covered the most common properties to style a web application and more importantly, what those styles do.
While there are more properties, more tools, and more innovations constantly being developed, you can always refer back to this article if you’re lost and need a refresher on the core topics of CSS.
If you work through each of these concepts during your intro to CSS and experiment with your own projects, you’ll become a CSS wizard in no time.
Read more
- How to Customize Content by Country with Shopify
- How to Manipulate Images with the img_url Filter
- How to Create Your First Shopify Theme Section
- Getting Started With a CSS Grid Layout on Shopify
- Free Webinar] Developing with Shopify: Using JavaScript to Add Ecommerce to Any Website
- 8 Free Resources For Learning Web Design and How to Code
- How Open Graph Tags can Optimize Social Media Experiences
- A Modern Approach to CSS Pt. 2: Building Resources
- 5 Easy-to-Implement Website Maintenance Tips for Your Client's Ecommerce Business
- Free Webinar] Getting Started with Sass and Shopify
What is your favorite CSS resource? Share your thoughts in the comments below!